Cheat sheets can be really helpful when you’re trying a set of exercises related to a specific topic, or working on a project. Because you can only fit so much information on a single sheet of paper, most cheat sheets are a simple listing of syntax rules. This set of cheat sheets aims to remind you of syntax rules, but also remind you of important concepts as well. You can click here and download all of the original sheets in a single document.
A more recently updated version of these sheets (April 2021) is available through Leanpub. The updated version includes a sheet that focuses on Git basics, a printer-friendly b&w version of each sheet, and each sheet as a separate document. There is an option to download the fully updated set at no cost.
We created this Python 3 Cheat Sheet initially for students of Complete Python Developer in 2021: Zero to Mastery but we're now sharing it with any Python beginners to help them learn and remember common Python syntax and with intermediate and advanced Python developers as a handy reference. There should be a subreddit just for Python cheat sheets. Everyone makes them yet no one needs them. 17 points 20 days ago. 4 points 20 days ago. Need one like this for c. We're working with Angular, and I have never touched Angular before, so its a whole new concept/architecture for me. Python re Examples Edit Cheat Sheet. For matching and replacing with regex patterns see Python re.sub’?, Python re.match’?, Python re.split’? Using re.
Re Cheat Sheet Python
If you’d like to know when more resources become available, you can sign up for email notifications here.
Overview Sheet
- Beginner’s Python Cheat Sheet
- Provides an overview of the basics of Python including variables, lists, dictionaries, functions, classes, and more.
Python Basics
- Beginner’s Python Cheat Sheet - Lists
- Focuses on lists: how to build and modify a list, access elements from a list, and loop through the values in a list. Also covers numerical lists, list comprehensions, tuples, and more.
- Beginner’s Python Cheat Sheet - Dictionaries
- Focuses on dictionaries: how to build and modify a dictionary, access the information in a dictionary, and loop through dictionaries in a variety of ways. Includes sections on nesting lists and dictionaries, using dictionary comprehensions, and more.
- Beginner’s Python Cheat Sheet - If Statements and While Loops
- Focuses on if statements and while loops: how to write conditional tests with strings and numerical data, how to write simple and complex if statements, and how to accept user input. Also covers a variety of approaches to using while loops.
- Beginner’s Python Cheat Sheet - Functions
- Focuses on functions: how to define a function and how to pass information to a function. Covers positional and keyword arguments, return values, passing lists, using modules, and more
- Beginner’s Python Cheat Sheet - Classes
- Focuses on classes: how to define and use a class. Covers attributes and methods, inheritance and importing, and more.
- Beginner’s Python Cheat Sheet - Files and Exceptions
- Focuses on working with files, and using exceptions to handle errors that might arise as your programs run. Covers reading and writing to files, try-except-else blocks, and storing data using the json module.
- Beginner’s Python Cheat Sheet - Testing Your Code
- Focuses on unit tests and test cases. How to test a function, and how to test a class.
Project-Focused Sheets
- Beginner’s Python Cheat Sheet - Pygame
- Focuses on creating games with Pygame. Creating a game window, rect objects, images, responding to keyboard and mouse input, groups, detecting collisions between game elements, and rendering text
- Beginner’s Python Cheat Sheet - Matplotlib
- Focuses on creating visualizations with Matplotlib. Making line graphs and scatter plots, customizing plots, making multiple plots, and working with time-based data.
- Beginner’s Python Cheat Sheet - Plotly
- Focuses on creating visualizations with Plotly. Making line graphs, scatter plots, and bar graphs, styling plots, making multiple plots, and working with geographical datasets.
- Beginner’s Python Cheat Sheet - Django
- Focuses on creating web apps with Django. Installing Django and starting a project, working with models, building a home page, using templates, using data, and making user accounts.
If you find any errors, please feel free to get in touch:
Email: ehmatthes@gmail.com
Twitter: @ehmatthes
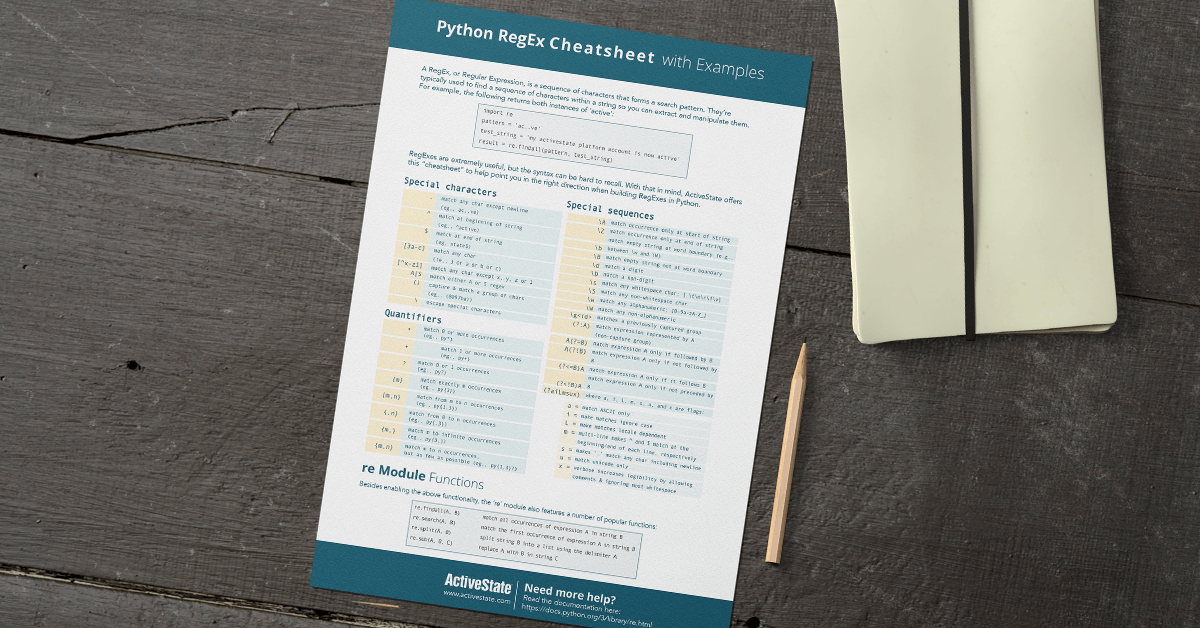
Above visualization is a screenshot created usingdebuggexfor the patternr'bpar(en|ro)?tb'
From docs.python: re:
Regular Expressions In Python
A regular expression (or RE) specifies a set of strings that matches it; the functions in this module let you check if a particular string matches a given regular expression
This blog post gives an overview and examples of regular expression syntax as implemented by the re
built-in module (Python 3.8+). Assume ASCII character set unless otherwise specified. This post is an excerpt from my Python re(gex)? book.
Elements that define a regular expression🔗
Anchors | Description |
---|---|
A | restricts the match to the start of string |
Z | restricts the match to the end of string |
^ | restricts the match to the start of line |
$ | restricts the match to the end of line |
n | newline character is used as line separator |
re.MULTILINE or re.M | flag to treat input as multiline string |
b | restricts the match to the start/end of words |
word characters: alphabets, digits, underscore | |
B | matches wherever b doesn't match |
^
, $
and are metacharacters in the above table, as these characters have special meaning. Prefix a
character to remove the special meaning and match such characters literally. For example,
^
will match a ^
character instead of acting as an anchor.
Feature | Description |
---|---|
| | multiple RE combined as conditional OR |
each alternative can have independent anchors | |
(RE) | group pattern(s), also a capturing group |
a(b|c)d is same as abd|acd | |
(?:RE) | non-capturing group |
(?P<name>pat) | named capture group |
. | Match any character except the newline character n |
[] | Character class, matches one character among many |
Greedy Quantifiers | Description |
---|---|
* | Match zero or more times |
+ | Match one or more times |
? | Match zero or one times |
{m,n} | Match m to n times (inclusive) |
{m,} | Match at least m times |
{,n} | Match up to n times (including 0 times) |
{n} | Match exactly n times |
pat1.*pat2 | any number of characters between pat1 and pat2 |
pat1.*pat2|pat2.*pat1 | match both pat1 and pat2 in any order |
Greedy here means that the above quantifiers will match as much as possible that'll also honor the overall RE. Appending a ?
to greedy quantifiers makes them non-greedy, i.e. match as minimally as possible. Quantifiers can be applied to literal characters, groups, backreferences and character classes.
Character class | Description |
---|---|
[aeiou] | Match any vowel |
[^aeiou] | ^ inverts selection, so this matches any consonant |
[a-f] | - defines a range, so this matches any of abcdef characters |
d | Match a digit, same as [0-9] |
D | Match non-digit, same as [^0-9] or [^d] |
w | Match word character, same as [a-zA-Z0-9_] |
W | Match non-word character, same as [^a-zA-Z0-9_] or [^w] |
s | Match whitespace character, same as [ tnrfv] |
S | Match non-whitespace character, same as [^ tnrfv] or [^s] |
Regex Cheat Sheet Pdf
Lookarounds | Description |
---|---|
lookarounds | custom assertions, zero-width like anchors |
(?!pat) | negative lookahead assertion |
(?<!pat) | negative lookbehind assertion |
(?=pat) | positive lookahead assertion |
(?<=pat) | positive lookbehind assertion |
(?!pat1)(?=pat2) | multiple assertions can be specified in any order |
as they mark a matching location without consuming characters | |
((?!pat).)* | Negate a grouping, similar to negated character class |
Flags | Description |
---|---|
re.IGNORECASE or re.I | flag to ignore case |
re.DOTALL or re.S | allow . metacharacter to match newline character |
flags=re.S|re.I | multiple flags can be combined using | operator |
re.MULTILINE or re.M | allow ^ and $ anchors to match line wise |
re.VERBOSE or re.X | allows to use literal whitespaces for aligning purposes |
and to add comments after the # character | |
escape spaces and # if needed as part of actual RE | |
re.ASCII or re.A | match only ASCII characters for b , w , d , s |
and their opposites, applicable only for Unicode patterns | |
re.LOCALE or re.L | use locale settings for byte patterns and 8-bit locales |
(?#comment) | another way to add comments, not a flag |
(?flags:pat) | inline flags only for this pat , overrides flags argument |
flags is i for re.I , s for re.S , etc, except L for re.L | |
(?-flags:pat) | negate flags only for this pat |
(?flags-flags:pat) | apply and negate particular flags only for this pat |
(?flags) | apply flags for whole RE, can be used only at start of RE |
anchors if any, should be specified after (?flags) |

Matched portion | Description |
---|---|
re.Match object | details like matched portions, location, etc |
m[0] or m.group(0) | entire matched portion of re.Match object m |
m[n] or m.group(n) | matched portion of nth capture group |
m.groups() | tuple of all the capture groups' matched portions |
m.span() | start and end+1 index of entire matched portion |
pass a number to get span of that particular capture group | |
can also use m.start() and m.end() | |
N | backreference, gives matched portion of Nth capture group |
applies to both search and replacement sections | |
possible values: 1 , 2 up to 99 provided no more digits | |
g<N> | backreference, gives matched portion of Nth capture group |
possible values: g<0> , g<1> , etc (not limited to 99) | |
g<0> refers to entire matched portion | |
(?P<name>pat) | named capture group |
refer as 'name' in re.Match object | |
refer as (?P=name) in search section | |
refer as g<name> in replacement section | |
groupdict | method applied on a re.Match object |
gives named capture group portions as a dict |
0
and 100
onwards are considered as octal values, hence cannot be used as backreferences.
re module functions🔗
Function | Description |
---|---|
re.search | Check if given pattern is present anywhere in input string |
Output is a re.Match object, usable in conditional expressions | |
r-strings preferred to define RE | |
Use byte pattern for byte input | |
Python also maintains a small cache of recent RE | |
re.fullmatch | ensures pattern matches the entire input string |
re.compile | Compile a pattern for reuse, outputs re.Pattern object |
re.sub | search and replace |
re.sub(r'pat', f, s) | function f with re.Match object as argument |
re.escape | automatically escape all metacharacters |
re.split | split a string based on RE |
text matched by the groups will be part of the output | |
portion matched by pattern outside group won't be in output | |
re.findall | returns all the matches as a list |
if 1 capture group is used, only its matches are returned | |
1+, each element will be tuple of capture groups | |
portion matched by pattern outside group won't be in output | |
re.finditer | iterator with re.Match object for each match |
re.subn | gives tuple of modified string and number of substitutions |
The function definitions are given below:
Regular expression examples🔗
As a good practice, always use raw strings to construct RE, unless other formats are required. This will avoid clash of special meaning of backslash character between RE and normal quoted strings.
- examples for
re.search
- difference between string and line anchors
- examples for
re.findall
- examples for
re.split
- backreferencing within search pattern
- working with matched portions
- examples for
re.finditer
- examples for
re.sub
- backreferencing in replacement section
- using functions in replacement section of
re.sub
- examples for lookarounds
- examples for
re.compile
Regular expressions can be compiled using re.compile
function, which gives back a re.Pattern
object. The top level re
module functions are all available as methods for this object. Compiling a regular expression helps if the RE has to be used in multiple places or called upon multiple times inside a loop (speed benefit). By default, Python maintains a small list of recently used RE, so the speed benefit doesn't apply for trivial use cases.
Python re(gex)? book🔗
Visit my repo Python re(gex)? for details about the book I wrote on Python regular expressions. The ebook uses plenty of examples to explain the concepts from the very beginning and step by step introduces more advanced concepts. The book also covers the third party module regex. The cheatsheet and examples presented in this post are based on contents of this book.
Use this leanpub link for a discounted price.
